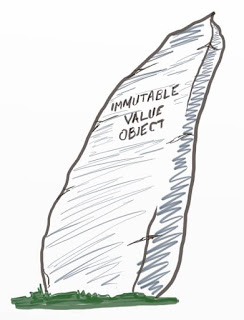
We need justification not to use immutable objects in our solutions, rather than an affirmative reason to use them. Records provide a first-class means for modeling data-only aggregates. They have been available in Java since the release of JDK 14.
Records are often seen as syntactic sugar to easily create immutable objects without having to write too much boilerplate code. Records as a clean definition of immutable objects can have a tremendous impact on your component design.
You as a designer have to reflect why your abstractions are not immutable and what is the rationale behind. From my experience, it improves the quality of your design.