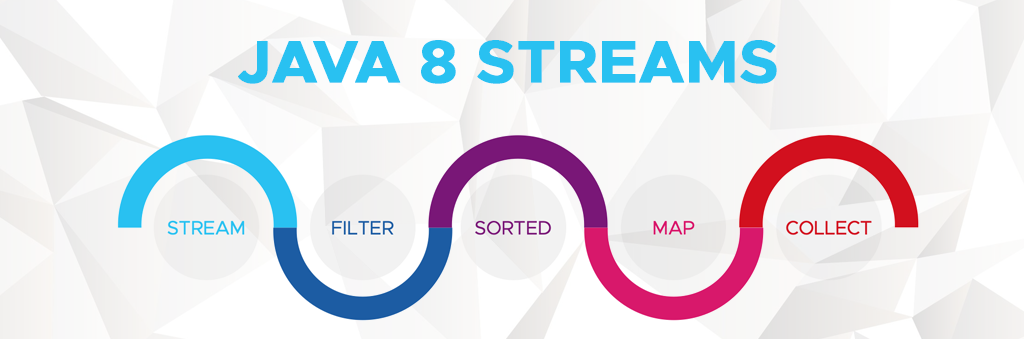
The Java Stream API is a powerful and simple to understand set of tools for processing sequences of elements. The standard collections were retrofitted with the stream() method, which allows us to convert any collection to a stream.
Modern Java code has almost no loops and conditional statements. It relies on the Stream API and the functional programming style to implement algorithms.