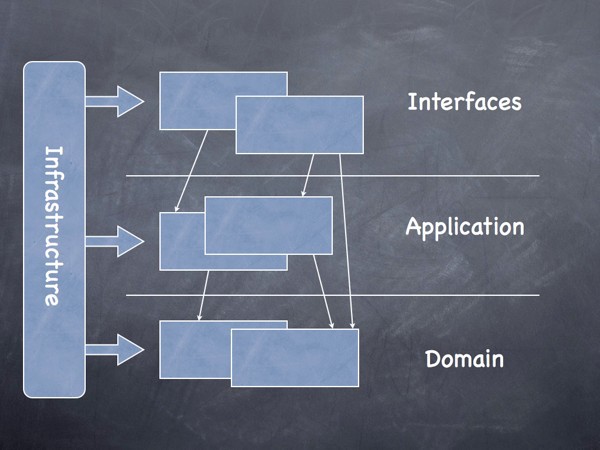
Domain Driven Development DDD is the preferred software architecture approach for designing digital products. The method nicely matches with the operational concepts of microservices.
Domain-driven design is a holistic approach to understanding, designing and building software applications.
Concrete recipes and examples of code structure are still sparse.