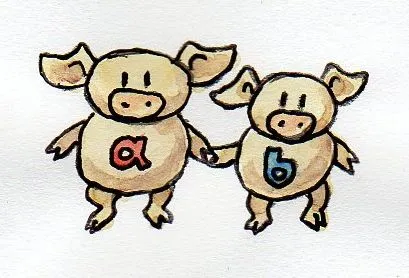
Records, sealed types, enumerations are the key language features for Algebraic Data Types.
The features have been available since Java 17.
Latter JDK releases provide additional capabilities and syntactic sugar.
Based on the features of JDK 22, we are still missing with constructors for records and primitive support for pattern matching [1].
Pattern matching capabilities empower the developer to implement complex algorithms in a compact and legible way.
Patterns such as visitor pattern are obsolete. You can write the same functionality with one pattern matching switch case, and the compiler would validate completeness for you.
The compiler checks the exhaustiveness of the pattern matching. Source code is more maintainable and less error-prone.