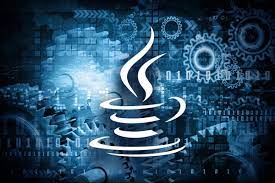
Java development activities have won traction since the decision to release a new version to the community every six months. Preview and incubator features promote feedback from the community and users.
Some tool builders, such as JetBrains are working proactively and provide support for JDK in developments.
Other tool maintainers such as JaCoCo are dreadfully behind the release cycle.
We learnt to deal with the new speed and how to tackle the laggards. Some experiences were quite painful.
Below our findings and our current focus on developing software products.