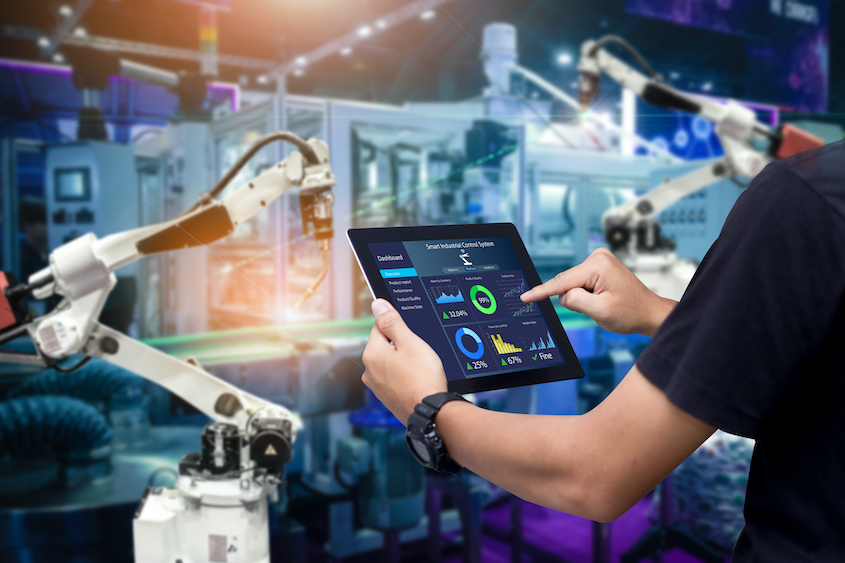
Embedded design maps domain abstractions to the underlying hardware.
A temperature sensor is a domain abstraction of a physical hardware temperature sensor.
A valve is a domain abstraction of a physical hardware valve. A physical valve can be a simple opened-closed device, or a proportional device.
The microcontroller board reads the temperature sensor through an I2C or SPI bus. It controls the valve through a GPIO, a PWM, or a CAN bus controller.
These hardware components map the domain object to the electronics controlling the physical device.