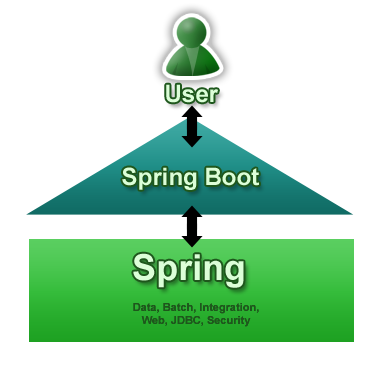
Spring and Spring Boot frameworks are the standard for enterprise Java applications.
Spring Boot makes it easy to create stand-alone, production-grade Spring-based Applications that you can run.
They take an opinionated view of the Spring platform and third-party libraries, so you can get started with minimum fuss.
Most Spring Boot applications need minimal Spring configuration.